Code for Top 20 Lists
Not too long ago, one of the members of the Pixel Pints shared with us a simple webapp that he found for building top-whatever lists, called Topsters.
I decided to play around with it a bit, and, as I mentioned in the last post—which was itself a top 20 list—I wanted to have an overlay for links on top of the image, so it was more like a widget instead of just an image. The power of Topsters is not the image that it generates necessarily, but how easily you can generate the image by querying a bunch of APIs based on your media selection (books, albums, movies, or games). So, in that regard adding a little flare to the image seemed appropriate, and why not give it some interactivity? Topsters itself utilizes a canvas element to arrange the pictures of the book, album, movie, or game covers. I could always consider maybe doing that myself, or
As I've mentioned, Write.as does give you a script area that you can use, and I don't think you can just inject JavaScript anywhere... I did try simply putting script tags in the markdown of a singular post, but it wasn't passing through when I inspected the live version of the site.
The next best option was to write an if statement which launches a specific function to act on a specific div when certain conditions are met.
You can clone from here, or simply take a look at the code:
//Global Variables to use:
const path = document.location.pathname;
/*--------------------------------------*/
function albumNum() {
let covers = document.getElementById("covers");
covers.style = "position: relative;";
for (let i = 1; i <= 20; i++) {
let numbers = `<a href="#item${i}"><p class="albNumber" id="cover-${i}">#${i}.</p></a>`;
covers.innerHTML += numbers;
setNumPos(i);
}
}
function setNumPos(num) {
let displayedNumber = document.getElementById(`cover-${num}`);
let imgHeight = covers.getElementsByTagName("img")[0].height;
console.log(imgHeight);
let imgWidth = covers.getElementsByTagName("img")[0].width;
console.log(imgWidth);
let row = [
imgWidth * 0.25,
imgWidth * 0.5,
imgWidth - imgWidth * 0.25
];
let col = [
imgHeight * 0.02,
imgHeight * 0.23,
imgHeight * 0.43,
imgHeight * 0.63,
imgHeight * 0.83
];
console.log("Widths " + row);
console.log("Heights " + col);
switch (num) {
case 1:
displayedNumber.style = `position: absolute; top: ${col[0]}px; left: 0px;`;
break;
case 2:
displayedNumber.style = `position: absolute; top: ${col[0]}px; left: ${row[0]}px;`;
break;
case 3:
displayedNumber.style = `position: absolute; top: ${col[0]}px; left: ${row[1]}px;`;
break;
case 4:
displayedNumber.style = `position: absolute; top: ${col[0]}px; left: ${row[2]}px;`;
break;
case 5:
displayedNumber.style = `position: absolute; top: ${col[1]}px; left: 0px;`;
break;
case 6:
displayedNumber.style = `position: absolute; top: ${col[1]}px; left: ${row[0]}px;`;
break;
case 7:
displayedNumber.style = `position: absolute; top: ${col[1]}px; left: ${row[1]}px;`;
break;
case 8:
displayedNumber.style = `position: absolute; top: ${col[1]}px; left: ${row[2]}px;`;
break;
case 9:
displayedNumber.style = `position: absolute; top: ${col[2]}px; left: 0px;`;
break;
case 10:
displayedNumber.style = `position: absolute; top: ${col[2]}px; left: ${row[0]}px;`;
break;
case 11:
displayedNumber.style = `position: absolute; top: ${col[2]}px; left: ${row[1]}px;`;
break;
case 12:
displayedNumber.style = `position: absolute; top: ${col[2]}px; left: ${row[2]}px;`;
break;
case 13:
displayedNumber.style = `position: absolute; top: ${col[3]}px; left: 0px;`;
break;
case 14:
displayedNumber.style = `position: absolute; top: ${col[3]}px; left: ${row[0]}px;`;
break;
case 15:
displayedNumber.style = `position: absolute; top: ${col[3]}px; left: ${row[1]}px;`;
break;
case 16:
displayedNumber.style = `position: absolute; top: ${col[3]}px; left: ${row[2]}px;`;
break;
case 17:
displayedNumber.style = `position: absolute; top: ${col[4]}px; left: 0px;`;
break;
case 18:
displayedNumber.style = `position: absolute; top: ${col[4]}px; left: ${row[0]}px;`;
break;
case 19:
displayedNumber.style = `position: absolute; top: ${col[4]}px; left: ${row[1]}px;`;
break;
case 20:
displayedNumber.style = `position: absolute; top: ${col[4]}px; left: ${row[2]}px;`;
}
}
if (path !=='/' && document.querySelector("#covers")) {
setTimeout(() => {
albumNum();
}, 500);
}
I need to do a bunch of stuff to this code; – re-write the switch statement as a function that checks for the number of rows and columns; and – re-write the for loop's iteration condition to be the rows multiplied by the columns.
I'm thinking I can signify by either reading the image file name, or using the id or class attributes.
The cool thing is that I can put it anywhere and the image will render.
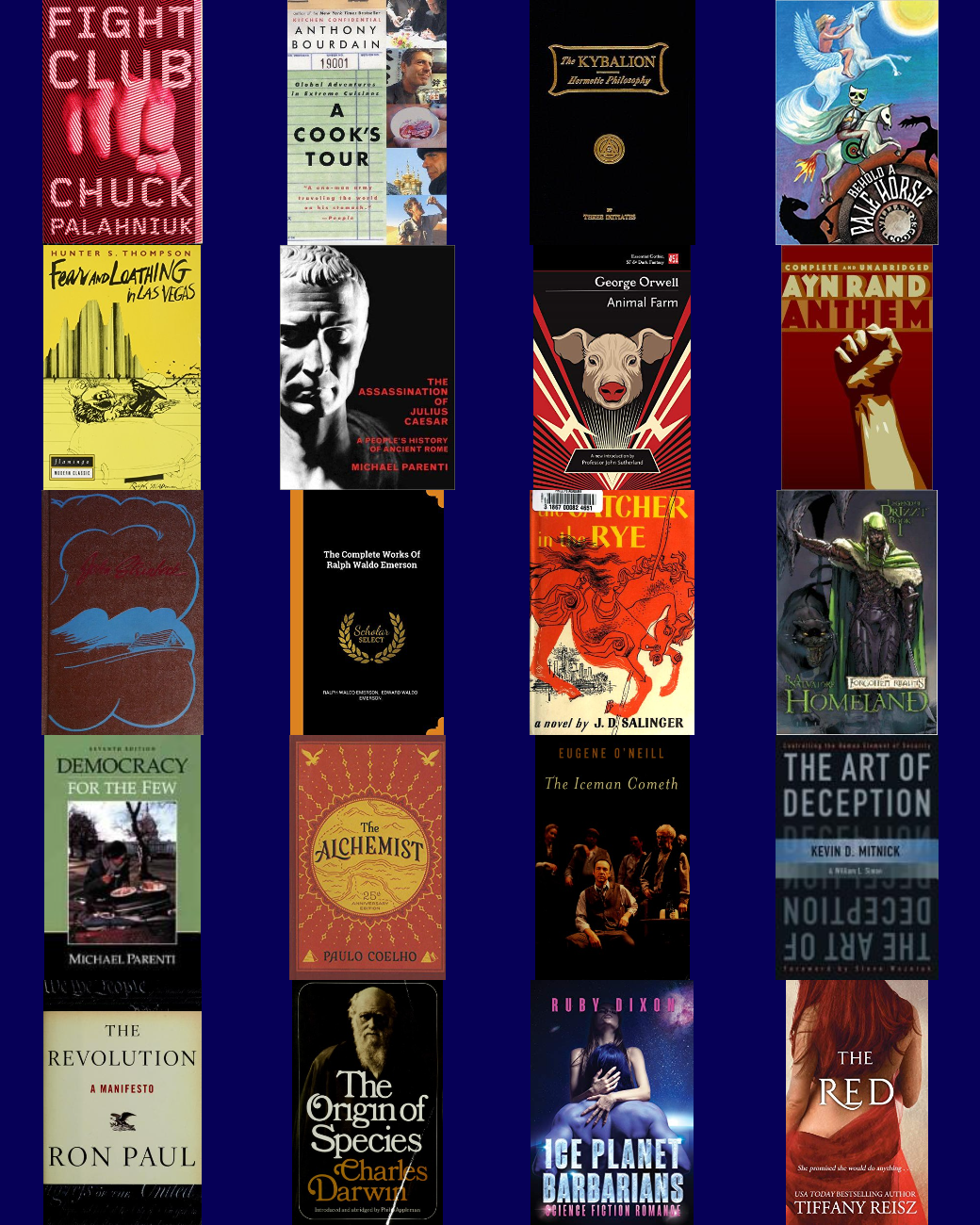